Drawing Shapes with Pygame
I wrote this in answering a question.
The Question
It should read from a file named shapes.txt
, in this format:
shapes.txt
:
circle 233 233 233 rectangle 125 211 32 line 21 21 21
Then render them with Pygame.
The Source
drawshapes.txt
:
1 #!/usr/bin/env python 2 # -*- coding: utf-8 -*- 3 4 import sys 5 from random import randint, seed 6 7 import pygame 8 from pygame.locals import * 9 10 SCREEN_X = 640 11 SCREEN_Y = 480 12 13 pygame.init() 14 15 def convert_strs_to_color(color_list): 16 return Color(int(color_list[0]), int(color_list[1]), int(color_list[2])) 17 18 def draw_circle(surface, color): 19 radius = randint(10, 100) 20 pos = (randint(radius, SCREEN_X-radius), randint(radius, SCREEN_Y-radius)) 21 pygame.draw.circle(surface, color, pos, radius, 1) 22 23 def draw_rectangle(surface, color): 24 height = randint(10, 100) 25 width = randint(20, 250) 26 left = randint(0, SCREEN_X-width) 27 top = randint(0, SCREEN_Y-height) 28 pygame.draw.rect(surface, color, (left, top, width, height), 1) 29 30 def draw_line(surface, color): 31 start_pos = (randint(0, SCREEN_X), randint(0, SCREEN_Y)) 32 while True: 33 end_pos = (randint(0, SCREEN_X), randint(0, SCREEN_Y)) 34 # make sure they are not on same spot 35 if end_pos != start_pos: 36 break 37 pygame.draw.line(surface, color, start_pos, end_pos) 38 39 opcode_dict = { 40 'circle' : draw_circle, 41 'rectangle' : draw_rectangle, 42 'line' : draw_line 43 } 44 45 print 'loading shapes.txt... ', 46 data = open('shapes.txt').readlines() 47 print 'ok' 48 print 'processing data... ', 49 tmp = [line.split() for line in data] 50 opcodes = [(line[0], convert_strs_to_color(line[1:])) for line in tmp if line] 51 print 'ok' 52 print 'initializing display... ', 53 screen = pygame.display.set_mode((SCREEN_X, SCREEN_Y), 0, 32) 54 screen.fill((0, 73, 156)) 55 print 'ok' 56 print 'initializing clock... ', 57 clock = pygame.time.Clock() 58 print 'ok' 59 print 'drawing shapes, press any key to quit...' 60 61 while True: 62 for event in pygame.event.get(): 63 if event.type in (QUIT, KEYDOWN): 64 sys.exit() 65 66 clock.tick(5) 67 68 for op in opcodes: 69 draw_func = opcode_dict[op[0]] 70 draw_func(screen, op[1]) 71 72 pygame.display.update() 73 74 print 'ok' 75 76 #* vim: set sts=4 sw=4 et fdm=marker: ********************* vim modeline **#
The Screenshot
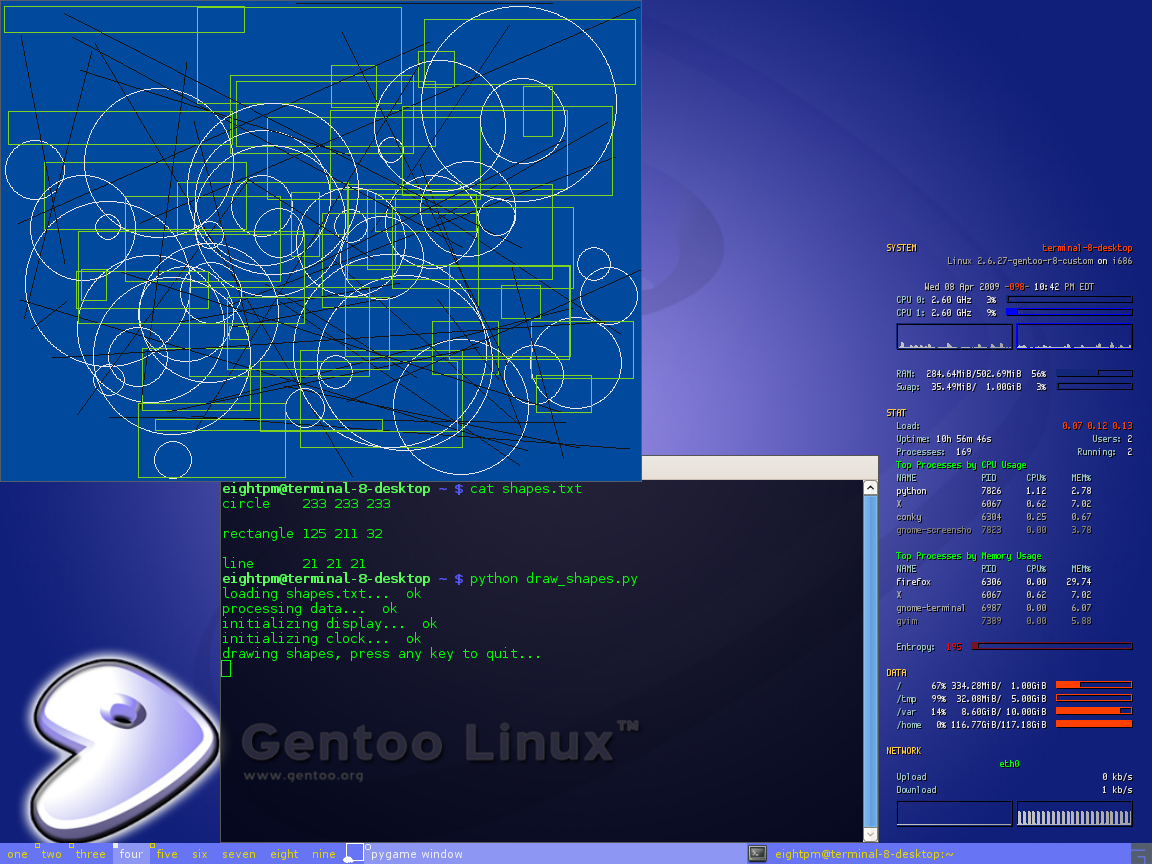